We are writing a program that will aid an elementary school student learns multiplication. Use random function to generate positive one digit integers and it should display questions of multiplication such as:
How much is 5 x 8 ?
The student then input the answer. The program should check the answer and response with correct or wrong message. Then another multiplication question is displayed. The process should be repeated until -1 is inputted by the student meaning to exit the program. Finally the program displays the number of correct answer and questions asked.
The program also should have three level of difficulties of question. The first level is multiplication of two integers. As the level goes up, the multiplication question consists of three integers and four integers for the highest level. The program should be able to adjust the level difficulty automatically according to the performance of the student. For example it should increase the level if the student able to answer correctly for five consecutive questions and vice-versa.
SOLUTION
PROGRAM ALGORITHM (FLOW OF PROGRAM)
1. Display user instructions
2. Display a multiplication question and compute the answer
3. Get data: user answer
4. Check the user answer with the computed answer
5. Determine the level of difficulty
6. Display the performance of the user
Steps 2-5 are repeated until the user exit the program.
PROGRAM DESIGN
We use functions to perform all required task in the program. There are six (6) functions have been defined accordingly, display the user instructions, generate integers, print the question, read user answer and check it with the correct answer, adjust the level of difficulty and display user performance.
Design of Function PrintUserInstructions
Input Parameter
None
Program Variable
None
Return Value
None
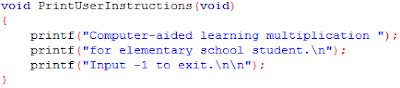
The definition consists of three statements to display user instructions.
Design of Function GenerateNumber
Input Parameter
None
Program Variable
None
Return Value
rand() % 9 + 1

The expression rand() % 9 + 1 always returns a value in the range 1 ≤ integer ≤ 9. Function rand() returns a pseudo-random number each time it is invoked. Pseudo-random number is a sequence of numbers that appears to be random that is generated using an initial value (seed). For a given seed, the sequence is always the same. If we use function rand() with the default seed, we will always get the same sequence. Therefore, in order to obtain a random number, we have to randomize the seed.
In C, there is a function called srand() that can be used to initialize the pseudo-random generator by supplying a seed value as an argument to it. Now what we have to do is to supply a different value each time the program is run. This can be achieved by using function time() which returns the number of seconds that have passed since 00:00:00 GMT January 1, 1970.
Design of Function PrintQuestion
Input Parameter
int level /* level of difficulty */
Program Variables
int i /* loop counter */
int num /*store generated integer */
int ans /* store the computed answer */
Return Value
int ans /* store the computed answer */
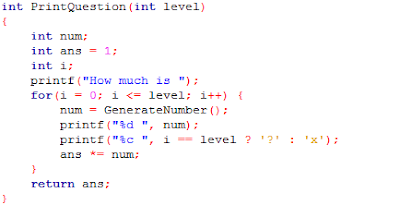
Function PrintQuestion uses for loop statement to print the multiplication question. In each iteration, an integer is generated by calling function GenerateNumber and multiplied with variable ans. The loop condition uses level (function's argument) in the loop condition. Initially, level has the value of 1 (level 1), therefore the loop is repeated twice which means the multiplication question will be consisted of two numbers.
Design of Function InputAnswer
Input Parameter
int correct_ans /* store the correct answer */
Program Variable
int user_ans /* store the user answer */
Global Variables
int cnt_question /* count the total questions have been asked */
int cnt_true /* count total true answer */
int cnt_consecutive_true /* count consecutive true answer */
int cnt_consecutive_false /* count consecutive false answer */
Return Value
QUIT 0
CONT 1
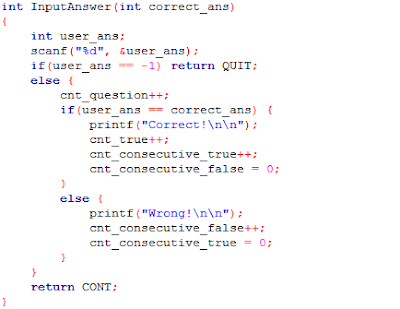
User answer is read by using scanf and stored in variable user_ans. Then user_ans is tested. If the value is -1, symbolic constant QUIT is returned. Else variable cnt_question is incremented and user_ans is tested. If user_ans is equal to correct_ans, variables cnt_true and cnt_consecutive_true are incremented and zero is assigned to cnt_consecutive_false.
Design of Function AdjustDifficulty
Input Parameter
int level
Program Variable
None
Return Value
int level
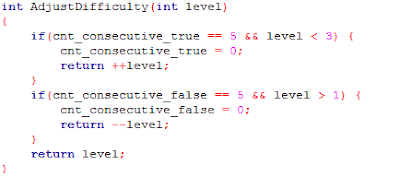
Difficulty level is adjusted based on the value of variables cnt_consecutive_true and cnt_consecutive_false.
Design of Function PrintResult
Input Parameter
None
Program Variable
None
Return Value
None
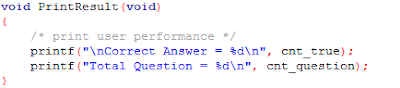
The definition consists of two statements to display the total number of correct answers and total number of questions asked.
IMPLEMENTATION
Begin by writing the #define directives for the constant data and global variables. In the main(), declare the variables for correct answer, level of difficulty and loop control variable. Then start coding each program algorithm steps. Call function PrintUserInstructions to display the user instruction/prompt. Then call the functions PrintQuestion, InputAnswer and AdjustDifficulty. These functions should be placed in the body of the program loop since they will be repeated until the user wants to exit. Finally call the function PrintResult to display the result. Don't forget to use comments in your program so as you won't get lost. Good luck and Happy Coding!
2 comments:
Thank you for sharing this example. It has been informative in a method of how to attain input in c coding. I will now explore further documentation on scanf(). Hopefully, it does support bounds checking, unlike gets().
Valuable info. Fortunate me I discovered your web site accidentally, and I am stunned why this accident didn't came about earlier! I bookmarked it.
Remove Pending Friend Request Facebook : Increase chance of not getting block
Post a Comment