Introduction
Lets revise what is a variable? A variable is a memory location that can store a value. An array is sequence of memory locations that have the same name and type. Thus we can say that an array is a collection of the same type of variables.
The following illustrates the graphical representation of an array.
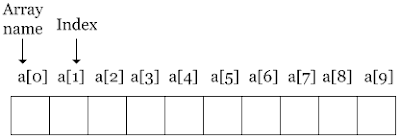
The array name is a and it has 10 elements. The name of an array can be declared like other variable names. It can contain letters, digit, underscore and cannot begins with digit. All the array's elements have the same data type, int, char or any legal type in C. Each element has its index number and we refer to an element using their index number. Thus if we want to access the fifth element for example, we refer it as a[4]. Notice that the first element of arrays start with zero.
So what is the usefulness of arrays? Arrays are used when we want to store information that have relation such as student's scores, item's prices etc. Of course we can use variables to store the student's scores such as follows.
int s1,s2,s3,s4,s5,s6,s7,s8,s9,s10;
printf("Enter 7 scores: \n");
scanf("%d%d%d%d%d%d%d%d%d%d",&s1,&s2,&s3,&s4,&s5,&s6,&s7,&s8,&s9,&s10);
This would work but what if we need to store 100 scores or 1000 scores. Its almost impossible to program such task. Well, this is where an array can be useful.
Defining and Initializing Arrays
Lets look at how to declare an array. Its like how we declare a variable except that we need to specify its size in square bracket.
int a[10];
Here we declare an integer array a that has 10 elements. We may define arrays to contain other data types such as char, float and double.
char a[10]; float a[10]; double a[10];
Now we know how to declare an array, lets looks at how to initialize it. The simplest way to initialize an array is as follows.
int a[10] = {32, 78, 64, 90, 27, 82, 18, 60, 94, 58};
Here we assign 32 to the the 1st element, 78 to the second element and so on. If there are fewer specified values than elements, the remaining elements are initialized to zero. For example,
int a[10] = {32, 78, 64, 90};
All elements of an array could have been initialized to zero as follows.
int a[10] = {0};
Now we know how to declare and initialize an array, lets look at an example. The program utilizes for statement structure to store 10 student's scores into an array.
Enter student's scores:
15
48
68
92
37
82
64
79
57
62
Your student's scores are:
15
48
68
92
37
82
64
79
57
62
Next: Character Arrays
Lets revise what is a variable? A variable is a memory location that can store a value. An array is sequence of memory locations that have the same name and type. Thus we can say that an array is a collection of the same type of variables.
The following illustrates the graphical representation of an array.
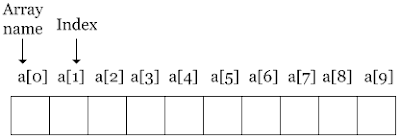
The array name is a and it has 10 elements. The name of an array can be declared like other variable names. It can contain letters, digit, underscore and cannot begins with digit. All the array's elements have the same data type, int, char or any legal type in C. Each element has its index number and we refer to an element using their index number. Thus if we want to access the fifth element for example, we refer it as a[4]. Notice that the first element of arrays start with zero.
So what is the usefulness of arrays? Arrays are used when we want to store information that have relation such as student's scores, item's prices etc. Of course we can use variables to store the student's scores such as follows.
int s1,s2,s3,s4,s5,s6,s7,s8,s9,s10;
printf("Enter 7 scores: \n");
scanf("%d%d%d%d%d%d%d%d%d%d",&s1,&s2,&s3,&s4,&s5,&s6,&s7,&s8,&s9,&s10);
This would work but what if we need to store 100 scores or 1000 scores. Its almost impossible to program such task. Well, this is where an array can be useful.
Defining and Initializing Arrays
Lets look at how to declare an array. Its like how we declare a variable except that we need to specify its size in square bracket.
int a[10];
Here we declare an integer array a that has 10 elements. We may define arrays to contain other data types such as char, float and double.
char a[10]; float a[10]; double a[10];
Now we know how to declare an array, lets looks at how to initialize it. The simplest way to initialize an array is as follows.
int a[10] = {32, 78, 64, 90, 27, 82, 18, 60, 94, 58};
Here we assign 32 to the the 1st element, 78 to the second element and so on. If there are fewer specified values than elements, the remaining elements are initialized to zero. For example,
int a[10] = {32, 78, 64, 90};
All elements of an array could have been initialized to zero as follows.
int a[10] = {0};
Now we know how to declare and initialize an array, lets look at an example. The program utilizes for statement structure to store 10 student's scores into an array.
#include <stdio.h> int main() { int i; int a[10]; printf("Enter student's scores: \n"); for(i = 0; i < 10; i++) { scanf("%d", &a[i]); } printf("Your students' scores are: \n\n"); for(i = 0; i < 10; i++) { printf("%d\n", a[i]); } return 0; }Sample output:
Enter student's scores:
15
48
68
92
37
82
64
79
57
62
Your student's scores are:
15
48
68
92
37
82
64
79
57
62
Next: Character Arrays
1 comment:
Nice work.That was very easy to understand,keep it up!
Post a Comment